Node Js Interview Questions
Node.js is a super popular server-side platform that more and more organizations are using. If you are preparing for a career change and have an upcoming job interview, it’s always a good idea to prepare and brush up on your interview skills beforehand. Although there are a few commonly asked Node.js interview questions that pop up during all types of interviews, we also recommend that you prepare by focusing on exclusive questions to your specific industry.
We have compiled a comprehensive list of common Node.js interview questions that come up often in interviews and the best ways to answer these questions. This will also help you understand the fundamental concepts of Node.js.
Question-1. What is Node.js? Where can you use it?
Answer- Node.js is an open-source, cross-platform JavaScript runtime environment and library to run web applications outside the client’s browser. It is used to create server-side web applications.
Node.js is perfect for data-intensive applications as it uses an asynchronous, event-driven model. You can use I/O intensive web applications like video streaming sites. You can also use it for developing: Real-time web applications, Network applications, General-purpose applications, and Distributed systems.
Question-2. Why use Node.js?
Answer- Node.js makes building scalable network programs easy. Some of its advantages include:
- It is generally fast
- It rarely blocks
- It offers a unified programming language and data type
- Everything is asynchronous
- It yields great concurrency
Question-3. How does Node.js work?
Answer- A web server using Node.js typically has a workflow that is quite similar to the diagram illustrated below. Let’s explore this flow of operations in detail.
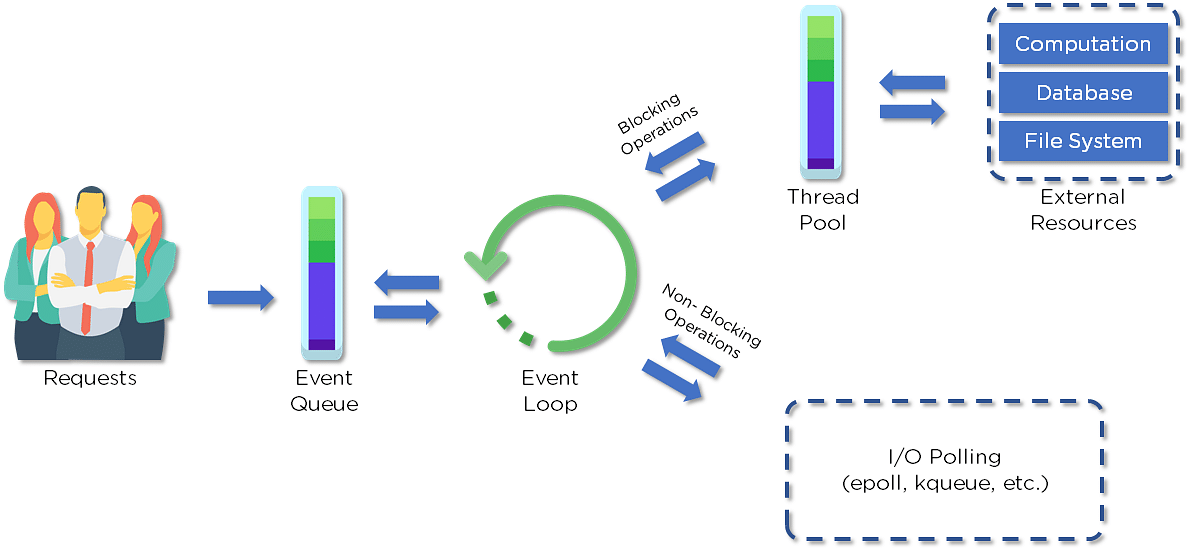
- Clients send requests to the webserver to interact with the web application. Requests can be non-blocking or blocking:
- Querying for data
- Deleting data
- Updating the data
- Node.js retrieves the incoming requests and adds those to the Event Queue
- The requests are then passed one-by-one through the Event Loop. It checks if the requests are simple enough not to require any external resources
- The Event Loop processes simple requests (non-blocking operations), such as I/O Polling, and returns the responses to the corresponding clients
A single thread from the Thread Pool is assigned to a single complex request. This thread is responsible for completing a particular blocking request by accessing external resources, such as computation, database, file system, etc.
Once the task is carried out completely, the response is sent to the Event Loop that sends that response back to the client.
Question-4. Why is Node.js Single-threaded?
Answer- Node.js is single-threaded for async processing. By doing async processing on a single-thread under typical web loads, more performance and scalability can be achieved instead of the typical thread-based implementation.
Question-5. If Node.js is single-threaded, then how does it handle concurrency?
Answer- The Multi-Threaded Request/Response Stateless Model is not followed by the Node JS Platform, and it adheres to the Single-Threaded Event Loop Model. The Node JS Processing paradigm is heavily influenced by the JavaScript Event-based model and the JavaScript callback system. As a result, Node.js can easily manage more concurrent client requests. The event loop is the processing model’s beating heart in Node.js.
Question-6. Explain callback in Node.js.
Answer- A callback function is called after a given task. It allows other code to be run in the meantime and prevents any blocking. Being an asynchronous platform, Node.js heavily relies on callback. All APIs of Node are written to support callbacks.
Question-7. What are the advantages of using promises instead of callbacks?
- The control flow of asynchronous logic is more specified and structured.
- The coupling is low.
- We’ve built-in error handling.
- Improved readability.
Question-8. How would you define the term I/O?
- The term I/O is used to describe any program, operation, or device that transfers data to or from a medium and to or from another medium
- Every transfer is an output from one medium and an input into another. The medium can be a physical device, network, or files within a system
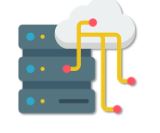
Question-9. How is Node.js most frequently used?
Answer- Node.js is widely used in the following applications:
- Real-time chats
- Internet of Things
- Complex SPAs (Single-Page Applications)
- Real-time collaboration tools
- Streaming applications
- Microservices architecture
Question-10. Explain the difference between frontend and backend development?
Front-end |
Back-end |
Frontend refers to the client-side of an application |
Backend refers to the server-side of an application |
It is the part of a web application that users can see and interact with |
It constitutes everything that happens behind the scenes |
It typically includes everything that attributes to the visual aspects of a web application |
It generally includes a web server that communicates with a database to serve requests |
HTML, CSS, JavaScript, AngularJS, and ReactJS are some of the essentials of frontend development |
Java, PHP, Python, and Node.js are some of the backend development technologies |